Did you know that JavaScript has been a programming language for 25 years?
We can attribute JavaScript’s continued success to the sheer power you can yield with it. Whilst many people might know what areas of a website you might find Javascript in, it is harder to answer the question “what does JavaScript even do?”
Thankfully, I’m here to tell you all about the different ways you can use JavaScript, through a very laboured metaphor.
For all of you Marvel fans out there, having an understanding of JavaScript is akin to Thanos wielding the infinity gauntlet with all six power stones in it.
Let me explain;
What powers do Thanos’s infinity stones grant him?
- Power Stone: The ability to destroy whole civilisations
- Space Stone: The ability to move through space through a wormhole
- Time Stone: The ability to alter and manipulate time
- Reality Stone: The ability to transform matter
- Mind Stone: The ability to manipulate the minds of others
- Soul Stone: The power over life and death
These powers give Thanos "near" limitless power when he combines them and there's very little that can stop him.
In the realm of JavaScript, there are no shiny gems, golden gloves or big purple men with a chin that looks like it got shut into a waffle machine, but what we do have are all of the same powers!
The POWER stone
Through the use of JavaScript, we gain the ability to destroy anything we want.
I'm not following, what can I destroy?
JavaScript is a "client-side" language, this means that all of its actions take place on the end-users’ machines, rather than on the server that the application is hosted on.
Why does this matter?
This is where our power comes from. With a server-side language, for example PHP, everything you see on a given web page happened BEFORE you saw it. Any calculations, any logic, anything that needed to happen, happened before the rendering of the page in your browser started.
JavaScript, however, doesn't (for the most-part) kick in UNTIL your page is loaded and continues to "listen" for as long as you remain on a given page.
This means that at any point we can trigger JavaScript to do something WITHOUT having to reload the page.
Let me give you an example of when we might want to flex our newly found power to destroy something...
If you're anything like me, I can't stand when I'm being force-fed adverts. Youtube quite often gives you something like this:
Usually, I'd just choose to ignore it, but now I wield the power stone, it would be rude not to make good use of it!
Let's say I create a quick Chrome extension (which basically runs Javascript on any web page in your browser).
Then I need to decide what the target of my wrath actually is.
To do this I'm going to right-click on the merch section in the web page, select "inspect" and it's going to open the console, which will show me the code behind this page.
Now it's a simple case of starting on the highlighted line that the browser has selected for us, and working upward until we find the top parent tag for this element (as we hover over each line the browser will highlight what is inside this element on the page itself, so keep going until you find the right line).
As you can see here, this line contains an ID named "merch-shelf".
Perfect, this means I can use my Javascript to target that parent element (and all of its children in true Thanos style).
All I need my Chrome extension to run is one very short line of code:
document.querySelector(“#merch-shelf").remove();
Now, every single Youtube page I visit, if the merchandise advertisement exists, my extension will destroy it for me so that I don't have to look at it, nice huh?
The SPACE stone
Sure, destroying stuff is nice. But it's not exactly ultimate power, is it?
No, for that, we need some other tricks up our sleeves.
The space stone grants Thanos the power to move through space in an instant without needing to travel between point A and point B.
What if I told you that we could transport data from somewhere else (another website even) and deposit it into our own script so that we can use it ourselves?
Pretty sweet I hear you say, believe it or not, this happens A LOT.
Developers use it on their own websites to get data from other server-side scripts on their own websites and load the data into the current page without having to reload or navigate elsewhere, and also to get data from third party services.
In fact, you might have heard about APIs (application programming interface). Their usage is widespread on the web and most massive services have one, Facebook, Spotify, Youtube etc.
Usually, what they do is output data from that particular service in a specific format (JSON, XML, etc), which you can query using your Javascript and “un-encode" the data you get back to give you nicely formatted Javascript objects that you can use in any way you choose.
It's quite complex what Javascript is actually doing to get this data for you, but in a nutshell, it runs an asynchronous request using something called an XMLHttpRequest.
This request will politely ask the destination server for some data whilst giving it some parameters to work with, and hopefully, this server will return to us what we asked for!
What we have now, is the ability to pull in WHATEVER WE WANT, from anywhere, to use on our page. That's already getting pretty powerful I'd say!
The TIME Stone
They say time stands still for no man.
What they don't say is that to a sufficiently JavaScript savvy man (or woman), time doesn't even exist.
This is all starting to get a bit trippy now, what am I talking about, time doesn't exist, good grief...
Hang in there, let me try and explain this in a way that makes sense to us as web users.
Currently in the year 2021, what is one of the most important factors on any website, before you even consider things like content, UI, UX etc?
Speed, right?
If you have the greatest website in the history of mankind but it takes an age to load, nobody is going to use it. We know this because of the emphasis people like Google give load time in their ranking algorithms.
Cast your mind back to earlier when I talked about server-side languages.
Well, you could consider HTML, CSS, PHP, etc to fit into that category, everything that you are seeing from these languages has already happened before the page loaded.
As a website becomes more feature-rich, naturally it will contain more resources (CSS, Javascript libraries), more complexity in the fetching of data (for example longer-running SQL queries), and before you know it, it’s going to start taking 3,4,5,10, maybe more seconds to load.
With each passing second, customers are being lost!
What can be done about this?
Let's fire up our time stone and see what benefit it can bring here.
The biggest offender when it comes to page load speed could be complex SQL queries that take some time to complete.
So rather than waiting for that, before we can render the page, what if we just loaded the page and pulled that data into the content AFTER the page had loaded?
You might have noticed a similar thing happening on sites like Facebook where you get greyed out "placeholder" posts before your actual news feed loads properly.
Can we take this a step further, what else can we load after the page has rendered (asynchronously)?
Of course we can. We can actually use JavaScript to load other JavaScript files. This is called "deferring" the script.
Often you will see a Javascript file being loaded as such:
In order to defer it, all we need to do is add the word "defer" to the script tag:
Excellent!
Anything else we can do?
Sure is, what about CSS, can we defer that as well?
We can, but it's not quite as simple as it was with JavaScript.
Normally, a CSS file would be loaded in the section of the HTML:
If we remove this however, and insert it via JavaScript we can save the time we would have had to wait for it to load, a bit like this:
var css = document.createElement('link');
css.href = '*url to our css document*';
css.rel = 'stylesheet';
css.type = 'text/css';
document.getElementsByTagName('head')[0].appendChild(css);
What will happen is that the page will load without the CSS, but then Javascript will insert it manually into the head for us when the page loads.
You probably will get a "flash of un-styled content", but this can be mitigated by a simple preloader animation.
So what's the benefit of doing this?
Instead of having to load all of these CSS and JS assets, and having to execute all of these time-consuming SQL queries before we can show a page, now we can get the page loaded MUCH faster, and pull in the content at our leisure.
This not only gives the user much better feedback, but also gives us much shorter response times for things like "first meaningful paint" and "time to interactive" in Google PageSpeed insights.
Which are obviously highly beneficial when it comes to not getting a ticking off from Google.
The REALITY Stone/The MIND Stone
For Thanos, the reality stone is something that can alter reality, or transform matter to anything he so desires.
He has been known to use it to create simulated realities that are indistinguishable from reality for the heroes he faces, in order to get what he wants.
This is where our foray into the world of JavaScript is going to take a rather dark turn I'm afraid.
Everything up until this point has been all sunshine and rainbows. It's all about helping us and making life better. But JavaScript can be used maliciously as well.
One such example of this is something called XSS (Cross-Site Scripting).
If we combine the metaphor of the reality stone with that of the mind stone, which allows Thanos to manipulate the minds of others, we might start to see some similarities with XSS.
Without getting needlessly complex, what this equates to is injecting a piece of Javascript code somewhere it doesn't belong on somebody else's website.
To do this, an attacker would need to find a vulnerability to allow them to inject the script and the methods that people use to find these are far outside the scope of this article, but just knowing that this is possible is enough for now.
When a malicious script is running on a website, the user has no way of knowing if what they are being shown is genuine or not, and as we discovered earlier, JavaScript is capable of changing just about anything.
In some cases, this might be used simply to send data that you insert into the web page to an alternate location rather than where you intended it to go. hink signing up for an account and sending your username and password to an attacker without even knowing it.
Or, it might take the form of downloading malicious files to your computer which can be exploited in other ways later on.
Whichever way you cut it, it's bad, and a fantastic example of how powerful JavaScript can be.
Just because you are on the right website, and you trust the owners, doesn't mean that you are 100% safe all of the times said website has been compromised.
The SOUL stone
The power that the soul stone grants Thanos is confusing at best, but it appears that it gives him control over life and death.
Furthermore, when it's combined with the power of the other stones, it gives him control over ALL life and death, allowing him to do his infamous "snap" which wiped out half of all life in the universe.
I like to think of this as control over the balance of natural order in the Marvel Universe. Having this control gives you ultimate power, there is nothing at this point you don't have control over.
The soul stone in a sense completes the power of all of the other stones.
However, with great power comes great responsibility.
The same applies directly to JavaScript.
You can use it to achieve damn near anything you want, it can create, destroy, change, request, send and generally manipulate anything and everything you could hope for. But it can prove incredibly difficult to debug sometimes.
For anybody coming from using a server-side language to using JavaScript, you are going to be in for a world of hurt as you begin to wrap your head around its asynchronous, wibbly-wobbly nature, the DOM, the virtual DOM, JavaScript frameworks such as jQuery, React, Angular, Vue and so on.
There are going to be times that you get an error which simply says, "there's been an error", as the JavaScript console decides you could afford to lose the rest of your evening as it trolls you hard.
Despite all of this, once you finally have that moment where everything falls into place, a new world of possibilities is going to open up for you and you too can wield the power of the infinity gauntlet.
When Thanos acquired the soul stone, he had to sacrifice what he loved to get it, in that he threw Gamora off a cliff (and he didn’t really spend that long thinking about it, harsh). I suppose what I’m saying to you is that to become a master of JavaScript, you are going to have to sacrifice everything you hold dear, friends, a social life, perhaps even personal hygiene. Is it worth it, hell yeah!
Wrap up
If you watched Endgame last year, you will have seen that the story in the film had played out 14,000,605 times until the heroes found a way to finally beat Thanos and save the day!
This provides us with the PERFECT metaphor for being a JavaScript user as you too will have to try things 14 million different ways until you find one that works. But how cool is it that there ARE 14 million different ways you could try something before exhausting all possibilities?
That just illustrates to us the sheer breadth of possibilities that JavaScript affords us.
It's been around for a long time and it'll be with us for a long time to come.
I bet there’s a load of stuff that you could use JavaScript for to make your job much easier.
And if you want to ask us more, get in touch or DM me on Twitter!
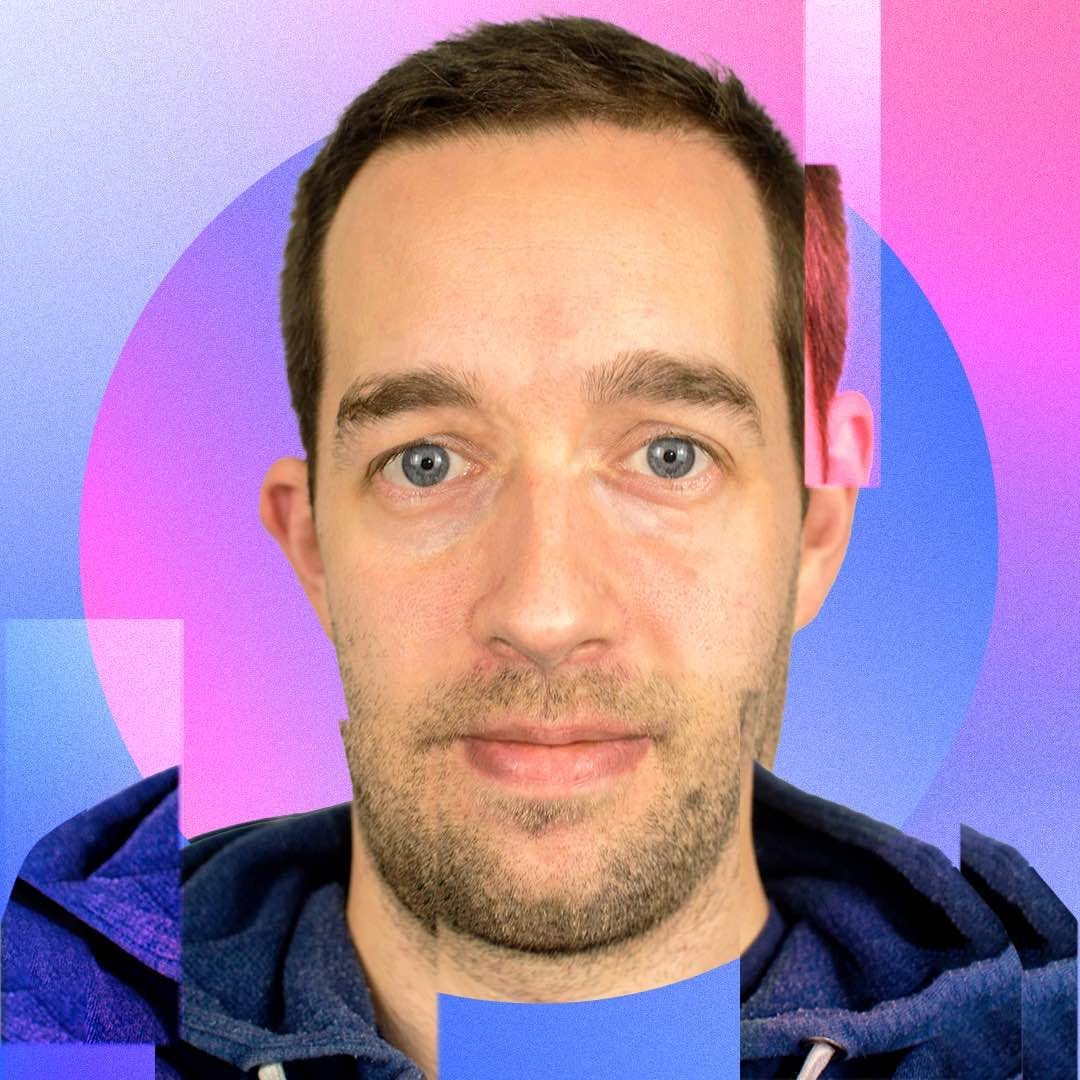